TLDR; drop the regex global option
The issue seems to be where consecutive lines include a match and it then ignores the even match lines.
Code
let ignoreResultsTaggedWith = "filter output";
//Added a match tag for my own testing
let includeResultsTaggedWith = "reg";
let searchScope = "inbox";
let modOrCreated = "created";
let searchStr = "@commit(";
let searchReg = /@commit\([^)]*\)/g;
let queries = "";
let drafts = Draft.query(searchStr, searchScope,[includeResultsTaggedWith],[ignoreResultsTaggedWith],modOrCreated,true);
drafts.map(theDraft =>
{
let hits = theDraft.lines.filter(element => searchReg.test(element));
alert("Matches = " + hits.length + "\n\n" + hits.join("\n"));
});
Draft
abc
def @commit(something alpha)
ghi @commit(something beta)
jkl @commit(something gamma)
mno @commit(something delta)
pqr
stu @commit(something theta)
If I modify the code to check the matching line by line to confirm the expectation of how it should match…
Code
let ignoreResultsTaggedWith = "filter output";
//Added a match tag for my own testing
let includeResultsTaggedWith = "reg";
let searchScope = "inbox";
let modOrCreated = "created";
let searchStr = "@commit(";
let searchReg = /@commit\([^)]*\)/g;
let queries = "";
let drafts = Draft.query(searchStr, searchScope,[includeResultsTaggedWith],[ignoreResultsTaggedWith],modOrCreated,true);
let astrOutput = [];
drafts.map(theDraft =>
{
theDraft.lines.map(theLine =>
{
astrOutput.push(searchReg.test(theLine) + "=> " + theLine);
});
});
alert(astrOutput.join("\n"));
… it shows non-matches for those even sequenced lines.
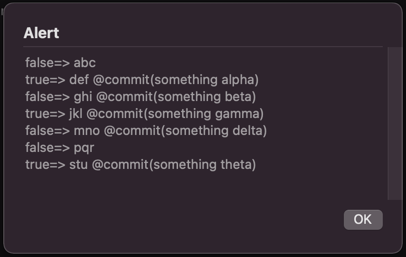
However, if I drop the g
for global matching…
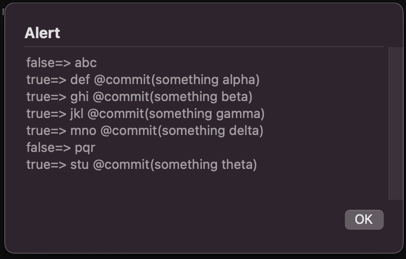
I think this might be necessaruy because we’re doing a true/false existence match rather than a full extraction of matching instances - though I don’t know the ensuing logic for why it would then give the ‘lost even’ results as seen.
So with a one character modification to my code at the top…
Code
let ignoreResultsTaggedWith = "filter output";
//Added a match tag for my own testing
let includeResultsTaggedWith = "reg";
let searchScope = "inbox";
let modOrCreated = "created";
let searchStr = "@commit(";
let searchReg = /@commit\([^)]*\)/;
let queries = "";
let drafts = Draft.query(searchStr, searchScope,[includeResultsTaggedWith],[ignoreResultsTaggedWith],modOrCreated,true);
drafts.map(theDraft =>
{
let hits = theDraft.lines.filter(element => searchReg.test(element));
alert("Matches = " + hits.length + "\n\n" + hits.join("\n"));
});
… I then get the following output, which is what I think we both would expect.
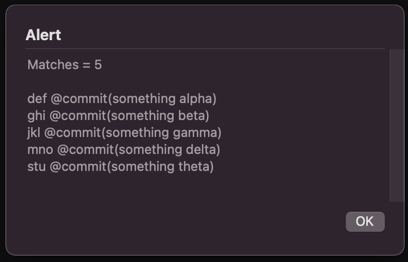
Hope that works for you too.
And I make it we have a little over two hours until our new year in the UK arrives, so hopefully, that’s in time too 